画面の一部をクリックしても、うまくボタンが反応しない時に、ボタンの上に何のオブジェクトが重なっているのかを調べます
コード
using System.Collections.Generic; using TMPro; using UnityEngine; using UnityEngine.EventSystems; using UnityEngine.UI; public class DebugLaycast : MonoBehaviour { [SerializeField]EventSystem eventSystem; [SerializeField]GraphicRaycaster raycaster; [SerializeField]TextMeshProUGUI textArea; void DetectUIElementsUnderMouse() { // マウス位置を取得 PointerEventData pointerData = new PointerEventData(eventSystem) { position = Input.mousePosition }; // ヒット結果を格納するリスト List<RaycastResult> results = new List<RaycastResult>(); // GraphicRaycasterを使用してレイキャスト raycaster.Raycast(pointerData, results); textArea.text = ""; if (results.Count > 0) { Debug.Log("Detected UI Elements:"); foreach (RaycastResult result in results) { textArea.text += result.gameObject.name + "\n"; // ヒットしたオブジェクト名をログに表示 // Debug.Log($"Hit UI Element: {result.gameObject.name}"); } } } // Update is called once per frame void Update() { // 左クリックしたとき if (Input.GetMouseButtonDown(0)) { DetectUIElementsUnderMouse(); } } }
オブジェクトと階層
任意の場所に設置できます
コンポーネント
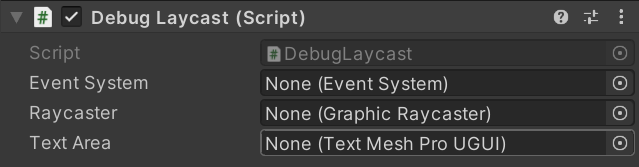
EventSystem
: イベントシステムを入れます
Raycaster
: 通常はCanvasに付いてますので、動作を確認したい Canvas をここに入れます
TextArea
: 画面上の見えやすい位置に TextMeshProUGUI を設置します。ここにクリック結果が表示されます
動作例
画面上をクリックする毎に、特定のキャンバス内のクリックしたポイントにある全ての Interactable なオブジェクトをテキストで表示してくれます。
クリックしても何か反応しないなと思ったときは、手軽に導入ができます。
(以上)